...
Code Block |
---|
language | java |
---|
theme | Midnight |
---|
|
package org.onap.demo.logging;
import javax.servlet.http.HttpServletRequest;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.onap.logging.ref.slf4j.ONAPLogAdapter;
import org.slf4j.LoggerFactory;
@Aspect
public class LoggingAspect {
@Before("execution(* org.onap.demo.logging.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
Object[] args = joinPoint.getArgs();
Object servletRequest = args[0];
ONAPLogAdapter.HttpServletRequestAdapter requestAdapter =
new ONAPLogAdapter.HttpServletRequestAdapter((HttpServletRequest)servletRequest);
final ONAPLogAdapter adapter = new ONAPLogAdapter(
LoggerFactory.getLogger(joinPoint.getTarget().getClass()));
try {
adapter.entering(requestAdapter);
} finally {
}
}
@After("execution(* org.onap.demo.logging.*.*(..))")
public void logAfter(JoinPoint joinPoint) {
final ONAPLogAdapter adapter = new ONAPLogAdapter(
LoggerFactory.getLogger(joinPoint.getTarget().getClass()));
adapter.exiting();
}
|
Logging Demo REST API
Code Block |
---|
|
curl http://dev.onap.info:30453/logging-demo/rest/health/health |
Logging Results
Use Case: Single REST call - with ENTRY/EXIT Markers around in-method log
The key here is that you get logs for free - the entry/exit lines are generated - the line in the middle is from java application code
Code Block |
---|
|
results - still working on passing in the servlet request
INFO: Reloading Context with name [/logging-demo] is completed
2018-07-09T14:48:01.014Z http-nio-8080-exec-8 INFO org.onap.demo.logging.ApplicationService
InstanceUUID=67bc4b12-56a1-4b62-ab78-0c8ca8834383,
RequestID=023af35a-b281-49c4-bf13-5167b1453780,
ServiceName=/logging-demo/rest/health/health,
InvocationID=94dc9e24-3722-43e5-8995-12f95e153ca3,
InvokeTimestamp=2018-07-09T14:48:01.008Z,
PartnerName=,
ClientIPAddress=0:0:0:0:0:0:0:1,
ServerFQDN=localhost ENTRY
2018-07-09T14:48:01.014Z http-nio-8080-exec-8 INFO org.onap.demo.logging.ApplicationService
InstanceUUID=67bc4b12-56a1-4b62-ab78-0c8ca8834383,
RequestID=023af35a-b281-49c4-bf13-5167b1453780,
ServiceName=/logging-demo/rest/health/health,
InvocationID=94dc9e24-3722-43e5-8995-12f95e153ca3,
InvokeTimestamp=2018-07-09T14:48:01.008Z,
PartnerName=,
ClientIPAddress=0:0:0:0:0:0:0:1,
ServerFQDN=localhost Running /health
2018-07-09T14:48:01.015Z http-nio-8080-exec-8 INFO org.onap.demo.logging.ApplicationService
ResponseCode=,
InstanceUUID=67bc4b12-56a1-4b62-ab78-0c8ca8834383,
RequestID=023af35a-b281-49c4-bf13-5167b1453780,
ServiceName=/logging-demo/rest/health/health,
ResponseDescription=,
InvocationID=94dc9e24-3722-43e5-8995-12f95e153ca3,
Severity=,
InvokeTimestamp=2018-07-09T14:48:01.008Z,
PartnerName=,
ClientIPAddress=0:0:0:0:0:0:0:1,
ServerFQDN=localhost,
StatusCode= EXIT |
...
Code Block |
---|
language | java |
---|
theme | Midnight |
---|
|
Tomcat v8.5 Server at localhost [Apache Tomcat]
org.apache.catalina.startup.Bootstrap at localhost:51622
Daemon Thread [http-nio-8080-exec-1] (Suspended (breakpoint at line 41 in LoggingAspect))
owns: NioEndpoint$NioSocketWrapper (id=147)
LoggingAspect.logBefore(JoinPoint) line: 41
NativeMethodAccessorImpl.invoke0(Method, Object, Object[]) line: not available [native method]
NativeMethodAccessorImpl.invoke(Object, Object[]) line: 62
DelegatingMethodAccessorImpl.invoke(Object, Object[]) line: 43
Method.invoke(Object, Object...) line: 498
AspectJMethodBeforeAdvice(AbstractAspectJAdvice).invokeAdviceMethodWithGivenArgs(Object[]) line: 629
AspectJMethodBeforeAdvice(AbstractAspectJAdvice).invokeAdviceMethod(JoinPointMatch, Object, Throwable) line: 611
AspectJMethodBeforeAdvice.before(Method, Object[], Object) line: 43
MethodBeforeAdviceInterceptor.invoke(MethodInvocation) line: 51
JdkDynamicAopProxy.invoke(Object, Method, Object[]) line: 213
$Proxy51.health(HttpServletRequest) line: not available
RestHealthServiceImpl.getHealth() line: 48
ServerRuntime$2.run() line: 326
WebComponent.service(URI, URI, HttpServletRequest, HttpServletResponse) line: 427
ServletContainer.service(URI, URI, HttpServletRequest, HttpServletResponse) line: 388
|
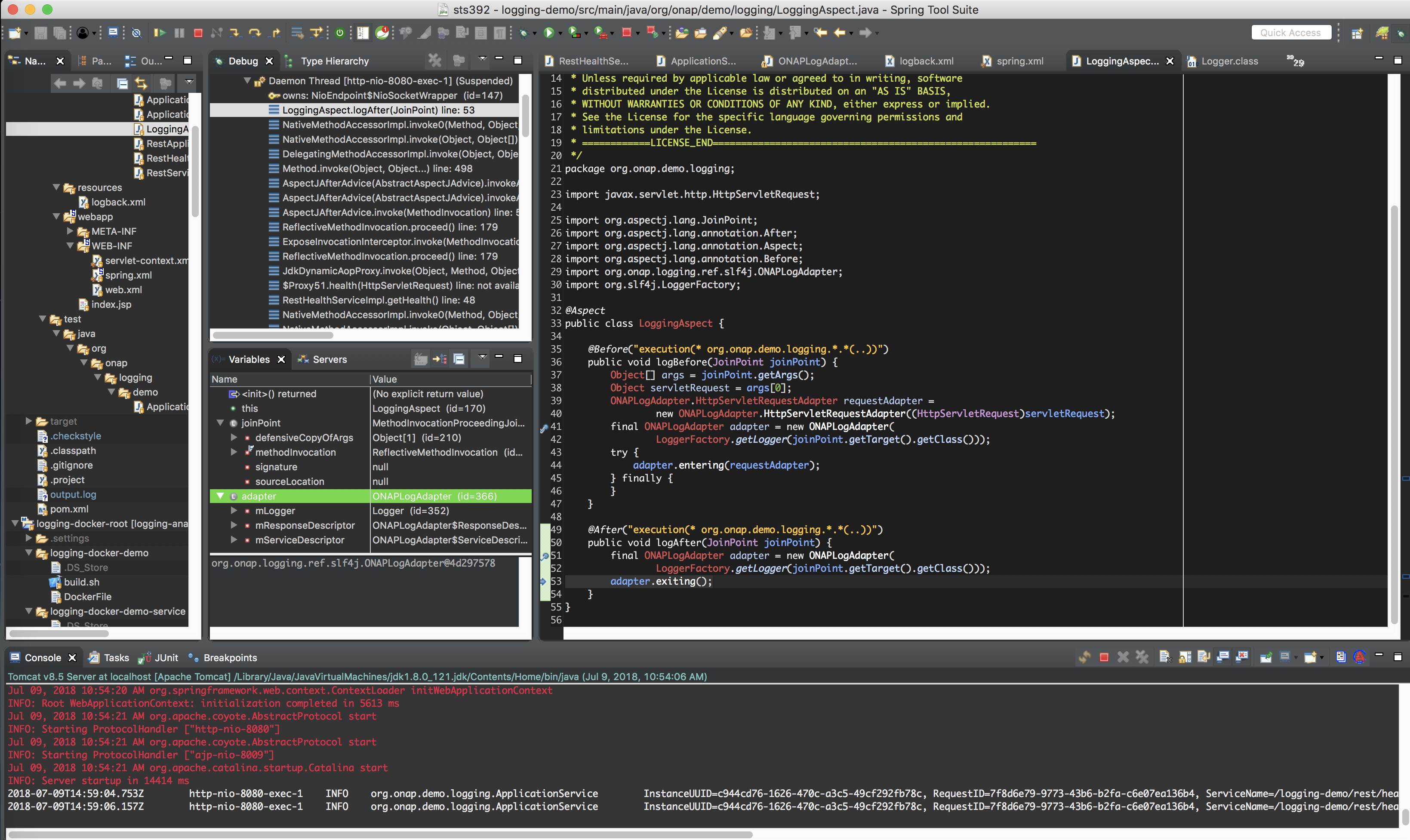
Deployment
Deploying demo pod
Helm Deployment
also as of 20180918 use Mike Elliott s plugin - https://gerrit.onap.org/r/#/c/67071/ at OOM Helm (un)Deploy plugins
...
Code Block |
---|
|
config:
logstashServiceName: log-ls
logstashPort: 5044 |
ELK Configuration
Logstash
Grok
Jira |
---|
server | ONAP JIRA |
---|
serverId | 425b2b0a-557c-3c0c-b515-579789cceedb |
---|
key | LOG-490 |
---|
|
ElasticSearch
Kibana
Design Issues
DI 8: Log Collection
...